- Go to Visual studio and Create a new Console Application Project
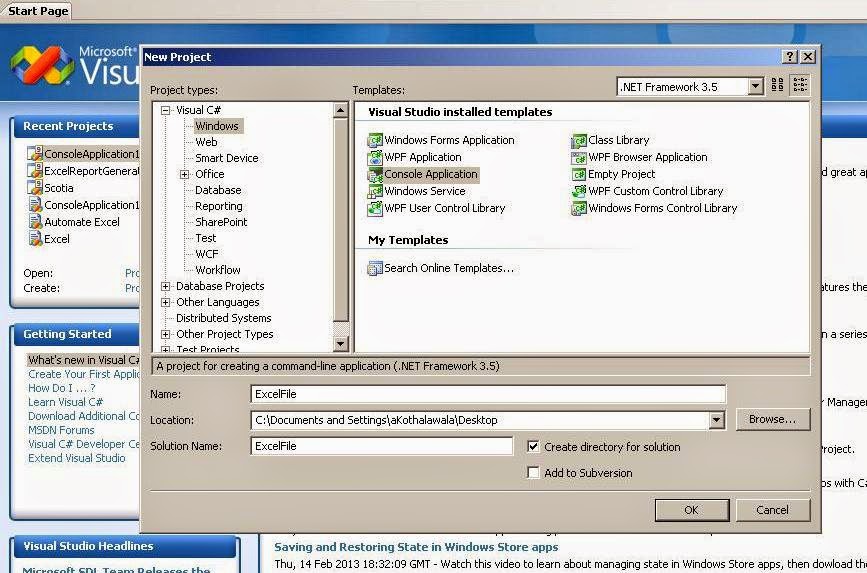
- To work with Excel related functions you have to add a com object called Microsoft Excel 14.0 Object Library in my case I'm using visual studio 2008 so it may be diffrent to you. but most of cases the reference name is same
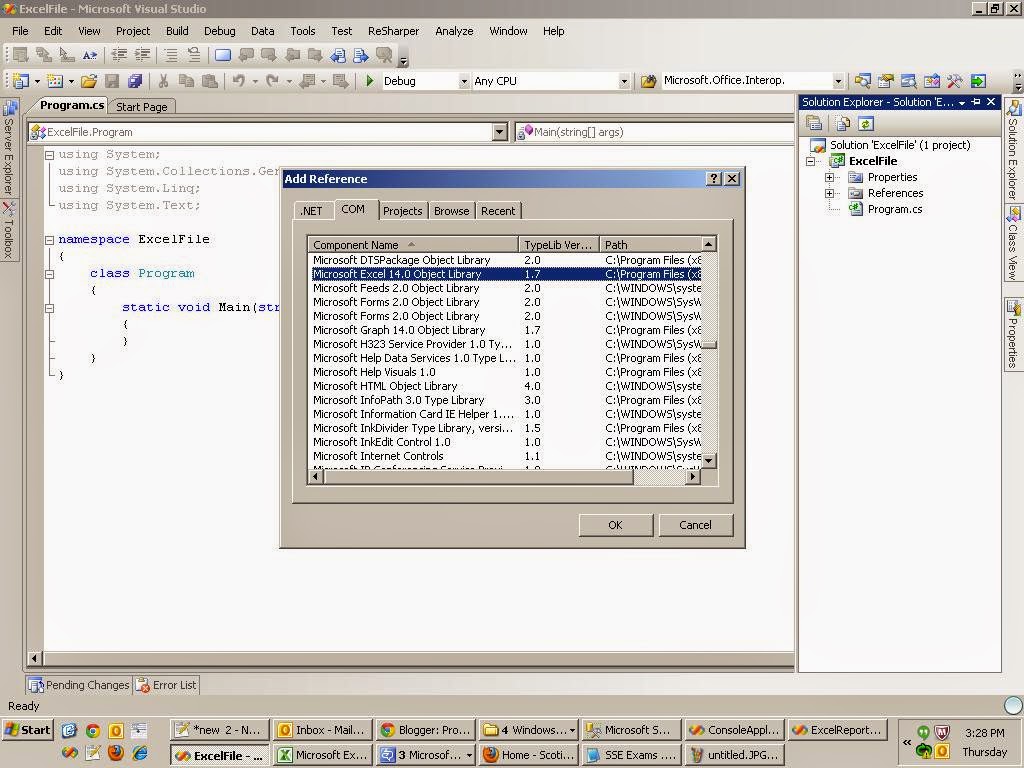

- Add following code segment to your Program.cs class and execute the program then you can see the newly created excel file on your Document folder. Happy coding.
using System;
using System.Data;
using System.Data.SqlClient;
using Excel = Microsoft.Office.Interop.Excel;
namespace ExcelFile
{
/// <summary>
/// Main Class
/// </summary>
class Program
{
/// <summary>
/// Main Methods
/// </summary>
/// <param name="args">Event Arguements</param>
static void Main(string[] args)
{
object misValue = System.Reflection.Missing.Value;
var excelApp = new Excel.ApplicationClass();
var excelWorkBook = excelApp.Workbooks.Add(misValue);
var excelWorkSheet = (Excel.Worksheet)excelWorkBook.Worksheets.get_Item(1);
excelWorkSheet.Cells[1, 1] = "Test Item Added";
//Save excel workbook
excelWorkBook.SaveAs("excel.xls", Excel.XlFileFormat.xlWorkbookNormal, misValue, misValue, misValue, misValue, Excel.XlSaveAsAccessMode.xlExclusive, misValue, misValue, misValue, misValue, misValue);
Console.WriteLine("File Created");
// close excel workbook
excelWorkBook.Close(true, misValue, misValue);
excelApp.Quit();
// --------- Important ---------------
// Release excel object when the process is over
ReleaseObject(excelWorkSheet);
ReleaseObject(excelWorkBook);
ReleaseObject(excelApp);
}
/// <summary>
/// Release Excel object when data writting is finish
/// </summary>
/// <param name="obj">Object which is going to relase</param>
private static void ReleaseObject(object obj)
{
try
{
System.Runtime.InteropServices.Marshal.ReleaseComObject(obj);
}
catch (Exception ex)
{
Console.WriteLine("Exception Occured while releasing object " + ex);
}
finally
{
GC.Collect();
}
}
}
}
Comments
Post a Comment