WCF (Windows Communication Foundation) for beginners
Hi guys after long time huh? so today i come up with a new topic but something very important to all of us. something you should really need if you are a great software engineer or a great programmer. so let's talk about WCF (windows communication foundation) by looking at a simple demo. because you can read lot of things regarding this topic in internet but the demos are kind of low to find. That's why I want to make this one a practical example. hope you understand.
1.so to create a WCF service or services you must create a new WCF project. (important run your visual studio as a administrator otherwise it'll not work) Go to visual studio and from Installed template select WCF and then select WCF Service library Project.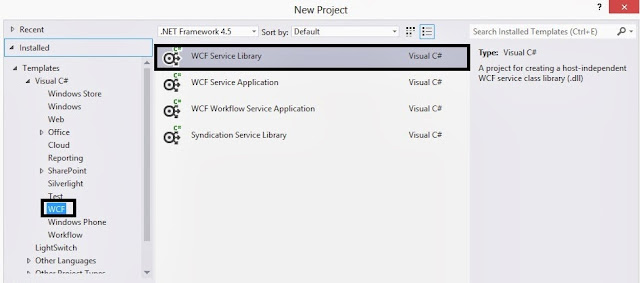
2. now give a proper name to the project. in here i'm going to give it a CalculationServices because this service is going to do simple calculation such as addition and multiplication.
3. Okay when you create a project it will give you some sample Service class and a interface. for our task we don't need it actually. so select both of them and delete them from the project.
4. Create a new Interface and name it as ICalculation and following method to it
5. Then add a new Class and name it as Calculation and Implement is using ICalculation interface
6. now we created the service it's time to configure it. so then others or even us can use that service. so go to the App.config file and paste it as it is or else change the things by your self (highly recommended).
okay we implemented the service then we configured it so now it's time to check the service is working properly. go and press F5. if you did it correctly you can see a window like below. if you got an error that mean you are not running your visual studio as an administrator or else there should be a some kind of mistake in your config file. please go and check them again
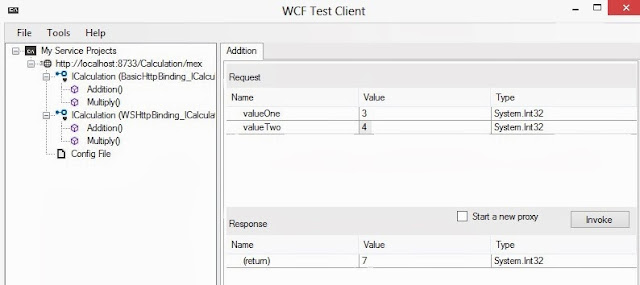
okay I teach you to create a very simple service using WCF and from next article I'll show how to connect the WCF application to a another project (Console, Window, Web). meanwhile you can try out some more in WCF for example try to implement a service using Data Contract and Data members which I never mention before. okay see you soon. bye bye
1.so to create a WCF service or services you must create a new WCF project. (important run your visual studio as a administrator otherwise it'll not work) Go to visual studio and from Installed template select WCF and then select WCF Service library Project.
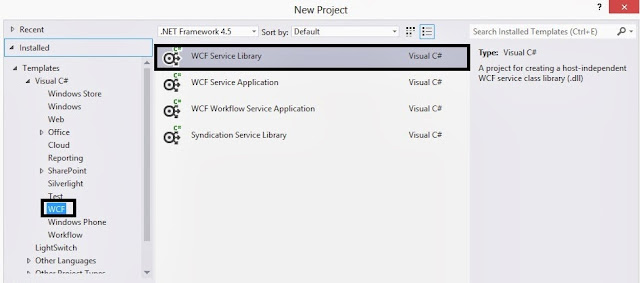
2. now give a proper name to the project. in here i'm going to give it a CalculationServices because this service is going to do simple calculation such as addition and multiplication.
3. Okay when you create a project it will give you some sample Service class and a interface. for our task we don't need it actually. so select both of them and delete them from the project.
4. Create a new Interface and name it as ICalculation and following method to it
#region Using Declaratives
using System.ServiceModel;
#endregion
namespace CalculationService.Interfaces
{
/// <summary>
/// ICalculation interface
/// </summary>
[ServiceContract]
public interface ICalculation
{
#region Public Methods and Operators
/// <summary>Add two values</summary>
/// <param name="valueOne">first value</param>
/// <param name="valueTwo">second value</param>
/// <returns><see cref="int"/></returns>
[OperationContract]
int Addition(int valueOne, int valueTwo);
/// <summary>Multiply two values</summary>
/// <param name="valueOne">first value</param>
/// <param name="valueTwo">second value</param>
/// <returns><see cref="int"/></returns>
[OperationContract]
int Multiply(int valueOne, int valueTwo);
#endregion
}
}
5. Then add a new Class and name it as Calculation and Implement is using ICalculation interface
#region Using Declaratives
using CalculationService.Interfaces;
#endregion
namespace CalculationService.Services
{
/// <summary>
/// CalculationServices Class
/// </summary>
public class CalculationServices : ICalculation
{
/// <summary>Add two values</summary>
/// <param name="valueOne">first value</param>
/// <param name="valueTwo">second value</param>
/// <returns><see cref="int"/></returns>
public int Addition(int valueOne, int valueTwo)
{
return valueOne + valueTwo;
}
/// <summary>Multiply two values</summary>
/// <param name="valueOne">first value</param>
/// <param name="valueTwo">second value</param>
/// <returns><see cref="int"/></returns>
public int Multiply(int valueOne, int valueTwo)
{
return valueOne * valueTwo;
}
}
}
6. now we created the service it's time to configure it. so then others or even us can use that service. so go to the App.config file and paste it as it is or else change the things by your self (highly recommended).
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<appSettings>
<add key="aspnet:UseTaskFriendlySynchronizationContext" value="true" />
</appSettings>
<system.web>
<compilation debug="true" />
</system.web>
<!-- When deploying the service library project, the content of the con-fig file must be added to the host's
app.config file. System.Configuration does not support con-fig files for libraries. -->
<system.serviceModel>
<services>
<service name="CalculationService.Services.CalculationServices">
<host>
<baseAddresses>
<add baseAddress = "http://localhost:8733/Calculation" />
</baseAddresses>
</host>
<endpoint address="" binding="basicHttpBinding" contract="CalculationService.Interfaces.ICalculation">
<identity>
<dns value="localhost"/>
</identity>
</endpoint>
<endpoint address="mex" binding="mexHttpBinding" contract="IMetadataExchange"/>
<endpoint address="ws" binding="wsHttpBinding" contract="CalculationService.Interfaces.ICalculation" />
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior>
<!-- To avoid disclosing meta-data information,
set the values below to false before deployment -->
<serviceMetadata httpGetEnabled="True" httpsGetEnabled="True"/>
<!-- To receive exception details in faults for debugging purposes,
set the value below to true. Set to false before deployment
to avoid disclosing exception information -->
<serviceDebug includeExceptionDetailInFaults="False" />
</behavior>
</serviceBehaviors>
</behaviors>
</system.serviceModel>
</configuration>
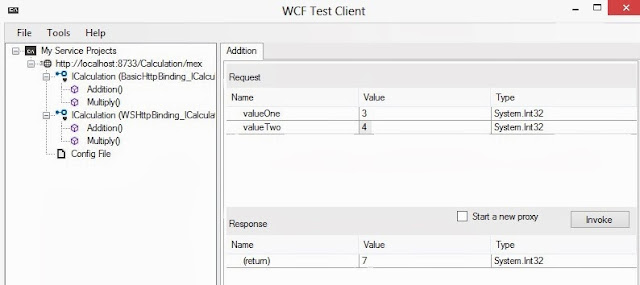
okay I teach you to create a very simple service using WCF and from next article I'll show how to connect the WCF application to a another project (Console, Window, Web). meanwhile you can try out some more in WCF for example try to implement a service using Data Contract and Data members which I never mention before. okay see you soon. bye bye
Comments
Post a Comment