How to Implement RESTful WCF Service
Windows Communication Foundation (WCF) is an SDK for developing and deploying services on Windows. WCF provides a runtime environment for your services, enabling you to expose CLR types as services, and to consume other services as CLR types. In this article, I am going to explain how to implement restful service API using WCF 4.0 . The Created API returns XML and JSON data using WCF attributes.
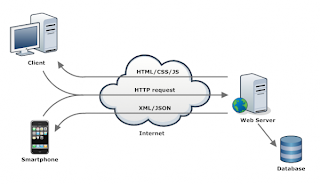
1. What is REST (Representational state transfer)
In simple form Representational state transfer (REST) is another cool architectural style. you can find more about the Restful architectural pattern here.
2. Why REST is famous these days.
- Less overhead (no SOAP envelope to wrap every call in)
- Less duplication (HTTP already represents operations like
DELETE
,PUT
,GET
, etc. that have to otherwise be represented in a SOAP envelope). - More standardized - HTTP operations are well understood and operate consistently. Some SOAP implementations can get finicky.
- More human readable and testable (harder to test SOAP with just a browser).
- Don't need to use XML (well, you kind of don't have to for SOAP either but it hardly makes sense since you're already doing parsing of the envelope).
- Libraries have made SOAP (kind of) easy. But you are abstracting away a lot of redundancy underneath as I have noted. Yes, in theory, SOAP can go over other transports so as to avoid riding a layer doing similar things, but in reality just about all SOAP work you'll ever do is over HTTP.
3. Implementation
3.1 Fist go to visual studio and select a new a new Project. Now then click on WCF Template and Select WCF Service Application. even you can select WCF Service Library but then you have to come-up with Host application to Host that WCF library.
3.2 You can delete both Service1.svc and IService1.cs Interface from the project and add a new WCF Service by right click on the project -> New Item -> WCF Service. then give a name whatever you like but in my case i'm going to name is as TestService. once you add WCF service you can remove default method from both interface and Service class
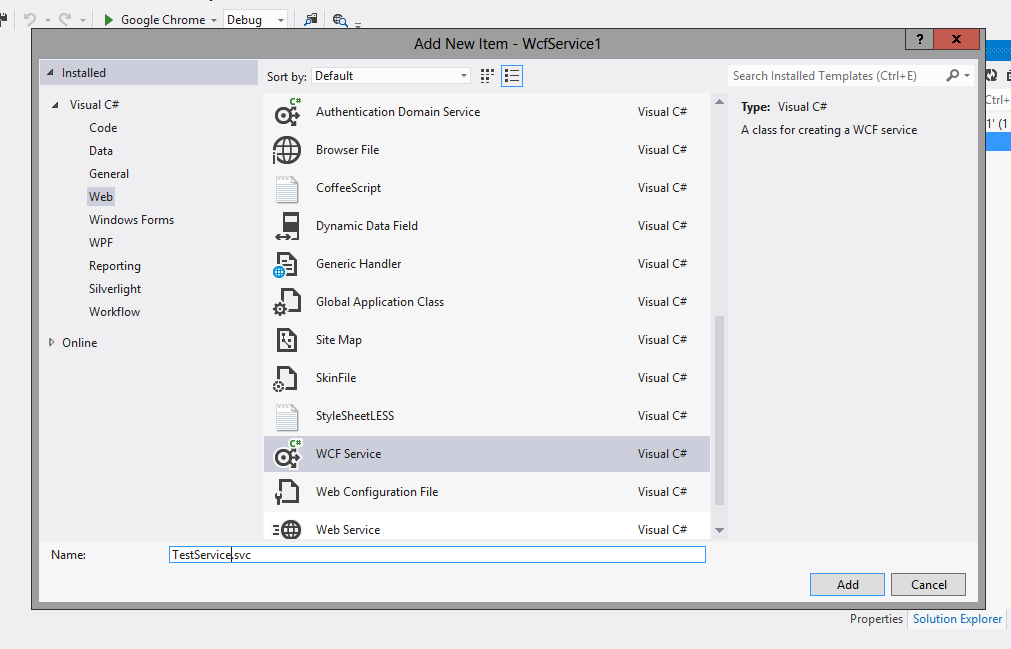
3.3 Okay now it's time to Implement the REST services. Here is the code segment I added to ITestService Interface.
[ServiceContract]
public interface ITestService
{
[OperationContract]
[WebInvoke(Method = "GET",
ResponseFormat = WebMessageFormat.Xml,
BodyStyle = WebMessageBodyStyle.Wrapped,
UriTemplate = "/GetNameXML?name={name}")]
string GetNameXML(string name);
[OperationContract]
[WebInvoke(Method = "GET",
ResponseFormat = WebMessageFormat.Json,
BodyStyle = WebMessageBodyStyle.Wrapped,
UriTemplate = "/GetNameJson?name={name}")]
string GetNameJson(string name);
}
3.3 And here is the code Implemtation for TestService class
public class TestService : ITestService
{
public string GetNameXML(string name)
{
return string.Format("Hello {0}", name);
}
public string GetNameJson(string name)
{
return string.Format("Hello {0}", name);
}
}
3.4 Okay now you are done with the Coding part. Now it's time to change the configuration file. below I added configuration changes which need to added to web.config file of the solution.
Note : All the changes are done inside <system.serviceModel> attribute. so don't change otherthings.
<system.serviceModel>
<services>
<service name="WcfService1.TestService" behaviorConfiguration="ServiceBehaviour">
<endpoint address="" binding="webHttpBinding" contract="WcfService1.ITestService" behaviorConfiguration="web">
</endpoint>
</service>
</services>
<behaviors>
<serviceBehaviors>
<behavior name="ServiceBehaviour">
<serviceMetadata httpGetEnabled="true"/>
<serviceDebug includeExceptionDetailInFaults="false"/>
</behavior>
</serviceBehaviors>
<endpointBehaviors>
<behavior name="web">
<webHttp/>
</behavior>
</endpointBehaviors>
</behaviors>
</system.serviceModel>
3.5 Great now you have cool Rest service which other can consume. Okay let's see whether it is working or not. press F5 on visual studio and now select the .svc file or if there is no errors then you can test it using your browser. here are the output of XML method and Json Method
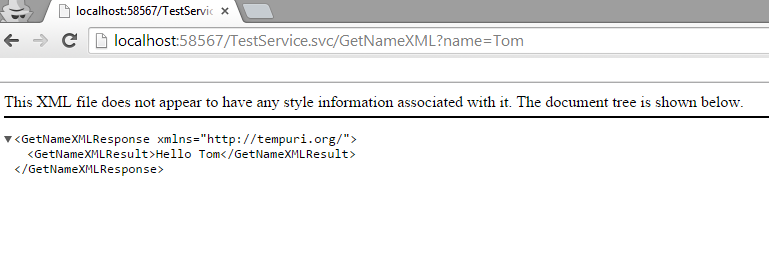
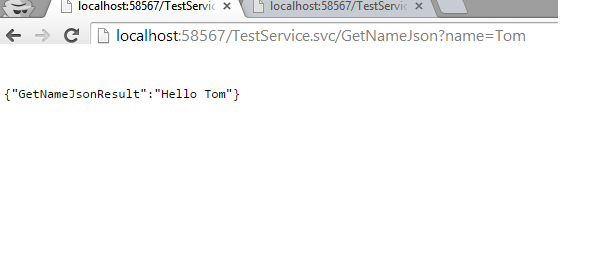
3.6 great now you completed your first REST service. like this you can implement complex methods and whatever you like. Happy coding guys. Have a nice day...!
Thanks for short and sweet article.
ReplyDelete